Lazy Loading in Angular: A Full Beginner’s Guide
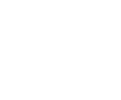
Angular, a widely-used JavaScript framework, excels in creating dynamic web apps, with lazy loading as a key feature enhancing efficiency and user experience. This technique allows loading specific application components only when needed, reducing initial load times and boosting performance. This beginner-friendly guide delves into lazy loading in Angular, exploring its significance and implementation in Angular applications.
Lazy loading in Angular, refers to the technique of loading website components, modules, or assets specifically when they are needed, rather than all at once. This approach is particularly relevant in Angular’s Single Page Applications (SPA), where traditionally, all components load simultaneously, potentially including unnecessary libraries or modules. While this might not significantly impact smaller applications, in larger applications, it can lead to increased load times. Thus, lazy loading optimizes performance by ensuring that components and modules are loaded only as required.
Quicker Page Loading | JavaScript, essential for displaying and loading your page, can slow things down because the browser needs to load all of it before showing your page. Lazy loading changes this by breaking JavaScript into chunks. The first chunk, which loads immediately, contains just what’s needed for the main module of your page. The rest of the modules load as they’re needed. This method speeds up how fast your page loads and appears. |
Reduced Data Usage | By breaking the data into chunks and loading them only, when necessary, your site can use less bandwidth. This is especially beneficial for users with limited data plans. |
More Efficient Browser Resource Use | Lazy loading means the browser only loads the chunks it needs, avoiding the use of memory and CPU on unneeded code. This makes for a more efficient use of browser resources. |
Learn more:
Here’s a must-have guide to implementing lazy loading in an Angular app.
Lazy Loading in Angular
This is essential for managing the angular lazy loading components within Angular 11’s modules.
To do this, use the command below to generate a module named ‘lazy-loading’ with its routing:
Step 1
Why start with a module? It’s because in Angular, you can’t lazy load individual components. This limitation stems from Angular’s architecture. Think of a module in Angular as a collection box that contains components, services, pipes, and more. It’s like a package that lists all the elements it contains and their dependencies.
In your application’s distribution, these dependencies come together to form a ‘chunk’ – essentially, a piece of transpiled JavaScript code. The main chunk includes modules that are directly imported, while those designated for lazy loading are grouped into a separate chunk. It’s important to note that while modules can form a chunk, individual components cannot. Hence, the inability to lazy load a single component.
In this phase, we’ll develop a lazy loading components angular module.
Take, for instance, a social media platform. Imagine there’s a route named ‘/user-dashboard’ that shows the user’s dashboard interface. This includes features like crafting a new post, viewing past posts, and other user activities. We’ll construct a component for this purpose and name it user-dashboard.
Use this command to create it:
Step 2
Place a link in the header for the route where lazy loading is to be applied. For instance, if you want the ‘user-dashboard’ component to be loaded only when its specific route is accessed, insert the following link in the header for the ‘/user-dashboard’ route.
Here’s the necessary code snippet for app.component.html:
Step 3
In this step, we implement lazy loading for the ‘/user-dashboard’ route using loadChildren. This can be done in two distinct methods:
Using Promise
Firstly, we explore the implementation of lazy loading Angular with loadChildren using a promise-based approach. To achieve this, modify the app-routing.module.ts as follows:
Step 4
Here, the ‘/user-dashboard’ route is specified in the path property. It’s important to note that the import statement also loads the lazy loading module we have created.
This code snippet employs a promise-based syntax to specify which routes should be lazily loaded. The routes intended for lazy loading are included in the loadChildren property as demonstrated. While promises are fundamental, more advanced versions like Angular 11 might favor a more streamlined coding approach.
Using Async
Alternatively, async can replace promises for a more straightforward, legible, and elegant code structure. The same functionality can be restructured using async as follows:
const routes: Routes = [
Step 4-2
The key distinction between this async-based code and the earlier promise-based one is the elimination of the .then() method. This is achieved by directly using the return value from await import(…), and directly referencing LazyLoadingModule in a single line, rather than employing then() and specifying LazyLoadingModule.
To finalize, we configure the route using the code below in the user-dashboard-routing.module.ts file.
Step 5
Great job! You’ve now successfully set up lazy loading in your Angular app. Whenever a user heads to a route needing the lazy-loaded module, it gets loaded just when needed. This enhances your app’s initial load time, making it more efficient.
There are several ways to implement lazy loading in angular, here are the most popular ones:
Organize your application into separate modules for each feature you plan to lazy load. This structure ensures that only the necessary code is loaded when required, enhancing performance.
Utilize the loadChildren method in your route configuration. This method allows Angular to load modules only when they are needed, significantly reducing the initial load time of the application.
Focus on reducing the size of your bundles and splitting your code effectively. This can be achieved by analyzing your bundles with tools like Webpack bundle analyzer, helping you identify and eliminate unnecessary code and dependencies.
Implement Lazy Loading in Angular
Adopt a strategy where each page of your application has its module, known as the Feature module. This approach simplifies your application’s structure and improves loading efficiency by isolating the code needed for each page.
Implement strategic preloading of modules. This involves preloading certain modules that users are likely to access next, based on their behavior. This strategy strikes a balance between immediate and lazy loading, improving user experience without compromising the initial load performance.
Continuously review and optimize the structure and loading behavior of your application. As your application grows and evolves, regular adjustments and optimizations are necessary to maintain and enhance the performance benefits gained from lazy loading.
In summary, lazy loading in Angular is a game-changer for enhancing application performance, especially for SPAs. It not only speeds up load times and reduces data usage but also optimizes browser resource utilization. However, effective implementation requires adherence to best practices like module organization, route configuration, and strategic preloading.
For those looking to leverage lazy loading in Angular or optimize existing projects, TECHVIFY offers expert services. Our team is well-versed in Angular best practices and can help you maximize your application’s efficiency and user experience. Reach out to TECHVIFY for top-notch Angular development support.
Table of ContentsI. What is Lazy Loading in Angular?II. Benefits of Lazy Loading in AngularIII. Implementing Lazy Loading in AngularStep 1: Begin by creating a module along with a dedicated routing file.Step 2: Create a Component Lazy Loading AngularStep 3: Insert the Link in the HeaderStep 4: Implement Lazy Loading with loadChildrenStep 5: Configuring the RouteIV. Best Practices for Implementing Lazy Loading in Angular1. Module Organization2. Route Configuration3. Bundle Size Reduction and Code Splitting4. One Module Per Page Strategy5. Strategic Preloading6. Regular Review and OptimizationConclusion GPT-4o is OpenAI’s third major iteration of their large multimodal model, expanding on GPT-4 with…
17 May, 2024
Table of ContentsI. What is Lazy Loading in Angular?II. Benefits of Lazy Loading in AngularIII. Implementing Lazy Loading in AngularStep 1: Begin by creating a module along with a dedicated routing file.Step 2: Create a Component Lazy Loading AngularStep 3: Insert the Link in the HeaderStep 4: Implement Lazy Loading with loadChildrenStep 5: Configuring the RouteIV. Best Practices for Implementing Lazy Loading in Angular1. Module Organization2. Route Configuration3. Bundle Size Reduction and Code Splitting4. One Module Per Page Strategy5. Strategic Preloading6. Regular Review and OptimizationConclusion Ask any leader of an enterprise-level company what’s most important, and they’ll likely say customers….
16 May, 2024
Table of ContentsI. What is Lazy Loading in Angular?II. Benefits of Lazy Loading in AngularIII. Implementing Lazy Loading in AngularStep 1: Begin by creating a module along with a dedicated routing file.Step 2: Create a Component Lazy Loading AngularStep 3: Insert the Link in the HeaderStep 4: Implement Lazy Loading with loadChildrenStep 5: Configuring the RouteIV. Best Practices for Implementing Lazy Loading in Angular1. Module Organization2. Route Configuration3. Bundle Size Reduction and Code Splitting4. One Module Per Page Strategy5. Strategic Preloading6. Regular Review and OptimizationConclusion A well-functioning web product is essential for startups. It can be a primary source of…
15 May, 2024
Thank you for your interest in TECHVIFY Software.
Speed-up your projects with high skilled software engineers and developers.
By clicking the Submit button, I confirm that I have read and agree to our Privacy Policy